Applying Zero Trust Principles to API Development: Security from the Ground Up

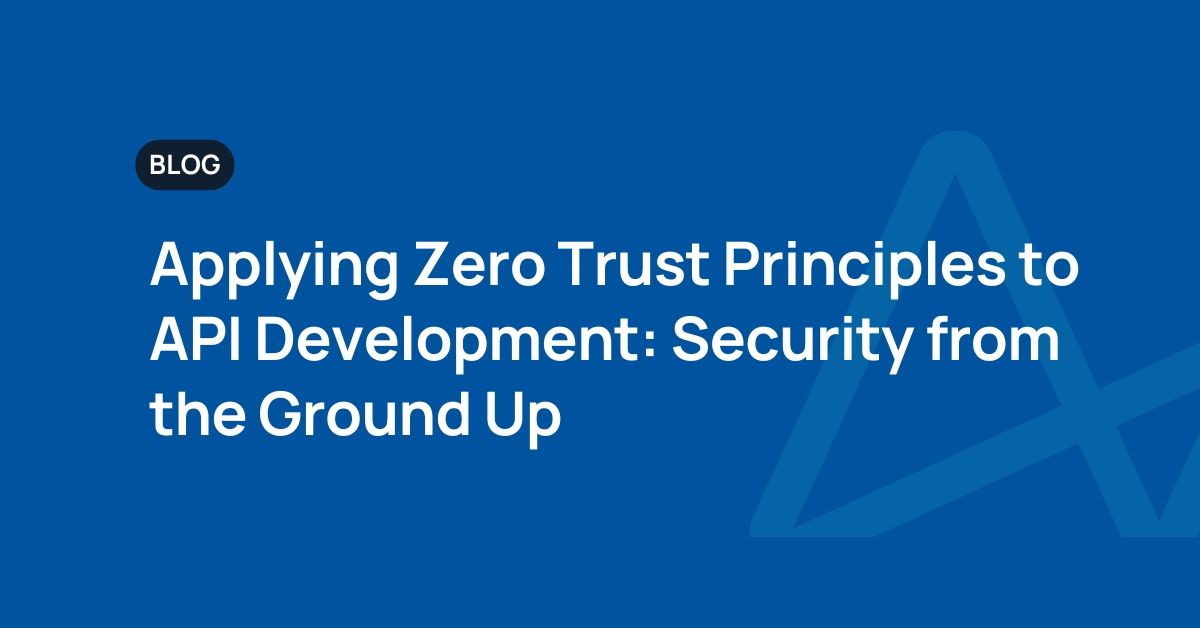
What are zero trust principles?
Core zero trust principles in API development
How Zero Trust Strengthens API Security
Implementing zero trust principles in API development
4 Best practices for APIs built on zero trust principles
Zero trust for event-driven or async APIs
Zero trust API maturity model
Think security, think zero trust
Security is essential. Whether you're building a physical entity like a house or a software product like a web application, if you don't factor in security, sooner or later, that system will be compromised. That's why it needs to be considered from the very beginning.
When it comes to implementing security in software applications, several techniques and principles are considered, such as encryption, authentication, authorization, and so on. However, one of the most effective and comprehensive approaches to security is the zero trust model.
This model is based on the principle of "never trust, always verify," meaning that no one, whether inside or outside the network, should be trusted by default. Instead, every request for access to resources must be verified and authenticated before being granted.
This article will explore the “ins and outs” of the zero trust model as it applies to API development. It will go over the core principles of the zero trust model, how to implement them in your API development process, and the benefits of doing so. By the end of this article, you will have a solid understanding of how to apply zero trust principles to your development process.
What are zero trust principles?
Zero trust principles are a set of ideas that guide how you secure systems by assuming that no one and nothing can be trusted without proof. It's a shift from the old way of thinking, where you might assume everything inside a network is safe once it's passed a perimeter check, like a firewall or authentication system.
With zero trust, every user, device, or piece of software trying to access a system has to show it's legitimate every single time it makes a move. This applies whether it's someone logging in from the office or a server connecting from halfway across the world. The core of zero trust is constant verification with the goal of ensuring that you know who or what is on the other end and that they're only doing what they're allowed to do.
Core zero trust principles in API development
In the context of API development, and even in the context of software development in general, zero trust principles can be broken down into three core principles: verify explicitly, least privilege access, and assume breach.
Let's take a closer look at each of these principles and how they can be applied to API development:
Verify explicitly
This principle is all about making sure every single request to your API proves itself, no exceptions. This means you don't just trust a request because it's coming from a familiar place or because it got through some initial check.
Every time someone or something tries to use your API, you demand clear, specific proof of who they are and what they're allowed to do. You might use tools like tokens or digital signatures that are tough to fake, and you validate them with every interaction.
It's a way to ensure that only the right people or systems get through, and you're never just hoping they're legit—you're confirming it outright.
Use least privilege access
This principle is all about keeping everyone's power as small as possible when they're interacting with your API. In practice, this means you only give users, devices, or applications the exact permissions they need to get their job done, and nothing more.
If someone's calling your API to read data, you don't let them write or delete anything—they're locked down to just reading. In API development, you set up these tight controls from the start, so even if someone sneaky gets in, they can't do much damage. It's all about limiting the potential “blast radius” by never handing out more access than absolutely necessary.
Assume breach
Assuming breach is a mindset that says, "Okay, we might get hacked, so let's act like it." This means you design your system with the idea that someone might get in, and you want to be ready for it.
You plan for the worst by adding extra layers of protection, like encrypting everything that moves through your API so it's useless if stolen or splitting up sensitive parts so no single break-in can ruin everything.
How Zero Trust Strengthens API Security
Zero trust principles are essential for API security because they provide a comprehensive framework for protecting sensitive data and resources. By implementing these principles, you can:
- Reduce the risk of data breaches: By verifying every API request and limiting access to only what is necessary, you can significantly reduce the risk of data breaches and unauthorized access to sensitive information.
- Enhance compliance: Many regulations and standards require strict access controls and data protection measures. Implementing zero trust principles can help you meet these requirements and avoid costly fines.
- Improve incident response: By monitoring and logging API usage, you can quickly identify and respond to security incidents, minimizing the impact of any breaches.
- Build trust with users: By demonstrating a commitment to security and data protection, you can build trust with your users and customers, enhancing your reputation and credibility in the market.
Implementing zero trust principles in API development
Implementing these principles requires a combination of technical measures, best practices, and a shift in mindset. Here are some key steps to consider:
- Authentication and authorization: Use strong authentication methods, such as OAuth 2.0 or OpenID Connect, to ensure that only authorized users can access your API. Implement fine-grained authorization controls to limit access based on user roles and permissions.
- Encryption: Use encryption to protect data in transit and at rest. This ensures that even if data is intercepted, it cannot be read without the proper keys.
- API gateway: Use an API gateway to manage and secure access to your APIs. An API gateway can provide features such as rate limiting, IP whitelisting, and logging to help monitor and control access to your APIs.
- Monitoring and logging: Implement API monitoring and logging to track API usage and detect any suspicious activity. This can help you identify potential breaches and respond quickly to any security incidents.
- Regular security audits: Conduct regular security audits and penetration testing to identify vulnerabilities in your API and address them before they can be exploited.
4 Best practices for APIs built on zero trust principles
Building an API with zero trust principles is a solid start, but keeping it secure over time takes ongoing effort and smart habits.
The best practices here are about making your API not just secure today but ready for whatever comes tomorrow. Here are some best practices to keep in mind:
1. Design with simplicity in mind
When you're designing an API with zero trust, keep it as simple as you can while still getting the job done. A complicated API with tons of API endpoints and sprawling permissions is harder to secure.
Stick to clear, focused endpoints that do one thing well, and make sure each one follows the same strict verification and privilege rules. This is about reducing the surface area an attacker could target. A lean API is easier to monitor, lock down, and fix if something goes wrong.
2. Automate security checks wherever possible
Nobody's perfect, and manually checking every part of your API for zero trust compliance is a recipe for mistakes. Lean on automation to handle repetitive tasks like validating tokens, scanning for outdated encryption, or flagging permission creep.
Set up tools that run these checks as part of your development pipeline so every update gets vetted before it goes live. Automation doesn't replace your judgment; it frees you up to focus on the big picture while catching the small slip-ups that could let an attacker in.
3. Prioritize clear documentation
Your API's security is only as strong as the people using it, so make sure everyone knows how to play by zero trust rules. Write API documentation that spells out exactly how to authenticate, what permissions to expect, and why things are locked down the way they are.
Don't just dump technical API specs —explain the reasoning, so developers understand why they can't skip steps or request extra access. Clear docs cut down on accidental misuse, which can be just as dangerous as an attack.
5. Rotate and refresh credentials regularly
Even the strongest keys get weaker if they sit around too long, so make it a habit to rotate your API's credentials on a schedule. Swap out tokens, update signing keys, and force new authentications periodically—don't let anything linger forever.
This practice limits the damage if a key gets stolen since it'll only work until the next refresh. It's a small effort that adds a big layer of protection, keeping your API one step ahead.
Zero trust for event-driven or async APIs
Event-driven or async APIs are a bit different from the traditional request-response model, but zero trust principles still apply. Here's how to adapt them:
- Event validation: Just like with regular API requests, every event should be validated. This means checking the source of the event, ensuring it's from a trusted producer, and verifying its integrity.
- Access control: Implement strict access controls for event consumers. Only allow authorized consumers to subscribe to events, and ensure they have the necessary permissions to process them.
- Audit logs: Maintain detailed audit logs of all events and their processing. This helps you track who accessed what and when making it easier to identify any suspicious activity.
- Rate limiting: Apply rate limiting to event producers and consumers to prevent abuse and ensure fair usage of resources.
- Data encryption: Encrypt sensitive data in events to protect them from unauthorized access. This is especially important for events that may be transmitted over public networks.
Zero trust API maturity model
The zero trust API maturity model is a framework that helps organizations assess their current level of zero trust implementation and identify areas for improvement.
It consists of four levels, each representing a different stage of maturity in applying zero trust principles to API development:
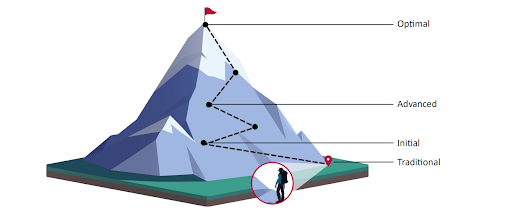
mage source: CISA's Zero Trust API Maturity Model
1. Traditional stage: At the traditional stage, you're still using the old-school way of doing things. You might have some basic authentication in place, but you're not really thinking about who gets access to what.
2. Initial stage: In the initial stage, you start to add some zero trust elements. You might use tokens that expire after a while, and you're beginning to think about who gets access to what. You're still not fully there, but you're on the right path.
3. Advanced stage: At this stage, you've got a solid zero-trust setup. You're using strong authentication methods and strict access controls, and you're constantly monitoring for suspicious activity. Your API is designed with security in mind from the ground up.
4. Optimal stage: In the optimal stage, you're not just secure—you're proactive. Your API assumes it's already compromised. Every request gets relentless checks, data is encrypted end-to-end, and access is granted only for the moment it's needed, then yanked back. Your API is split into isolated pieces so one break doesn't ruin everything.
Think security, think zero trust
The security of your API is not just a box to check off; it's a continuous process that requires constant vigilance and adaptation. By applying zero trust principles to your API development process, you can ensure that your APIs are secure right from the start.
In this article, we have explored the core principles of zero trust, how to implement them in your API development process, and the benefits of doing so. By following these principles and best practices, you can confidently build APIs that are not only secure but also resilient to potential attacks.